Loops are required when you want the same piece of code to run
again and again till a condition becomes true. In a loop therefore you
need a counter to check the condition. JavaScript supports two types of
loops:
·
For loop: The For loop in JavaScript should be used when
you need to perform certain initializations, then checks the condition,
and enters the loop only after the condition is true. The counter or the
other update statements are executed first time after the loop is executed
once and then the condition is evaluated again. The loop executes till
the condition returns 'false' and the loop stops.
Syntax
for (Initialization statements; Condition; Updation
statements)
{
Statements...
}
Example
<html>
<body>
<script type="text/javascript">
// This loop prints the table of four
for (var num1=0;num1<=10;num1++)
{
document.write("4X",num1,"=",4*num1,"<BR>");
}
</script>
</body>
</html> |
·
While loop: The While loop in JavaScript should be used
when you need to execute a piece of code for an undetermined number of
times. This loop can do all that a For loop can do but in a cluttered
way. This loop checks for a condition and enters the loop if the condition
is true and executes the loop till the condition returns 'false'. This
loop also allows you to use the break and continue
statements within loop.
Syntax
while (condition)
{
.statements...
}
Example
<html>
<body>
<script type="text/javascript">
// This loop prints the table of four
var num1 = 0;
while (num1 <= 10)
{
document.write("4X",num1,"=",4*num1,"<BR>");
num1++;
}
</script>
</body>
</html> |
Result of both the loops
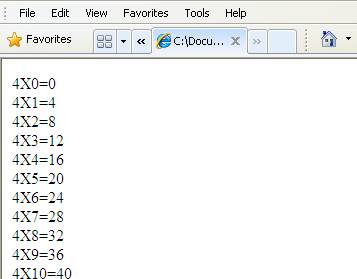
|