The web servers
cannot store the information or the history of the user. Once the browser
request has been fulfilled the information about the browser’s previous
actions will be lost. For example, the information such as which page
was clicked, or which image was clicked will be lost. The web server cannot
maintain the state of the user session. However, the maintaining the state
of the user is desirable most of the times and therefore cookies are used.
Cookie is
a variable that stores the information about the user session. It can
store the name, date, or the pages being visited by the user. The cookies
are stored on the user’s computer and when the user requests the same
page from the same browser, the cookie is sent to the server and help
server to identify the user and displays the name or the other information
of the user on the web page requested. There are different types of cookies
such as name cookies - that store the name of the user and helps in displaying
a welcome message for the user, password cookies - that stores the passwords
of the user and saves user from typing the password every time the user
visits the same website, date cookies – that stores the date and time
of the visit on the web page of the user and helps in displaying the last
visit date and time of the user.
The cookies
are however, expired after certain time. After which they will no longer
be stored at the user’s computer. JavaScript allows you to create and
read a cookie. To create a cookie, you need to use the code:
Document.cookie
= ”name=<cookiename>;expires=< expirydate>;path=<path>;domain=<domain>;secure”; |
The description
of each property is as follows:
·
Name= Name of the cookie.
·
Expires= Expiry date of the cookie in format weekday ddmmyy hh:mm:ss GMT.
By default the expiry date is set to the end of the current session.
·
Path= The directory path where the cookie is active or the portion of
the URL for which cookie is valid. Usually the path is set to “/”which
means the cookie is valid during the entire program.
·
Domain name= Domain portion of the URL for which the cookie is valid.
·
Secure = Specifies that the cookie should be transmitted over a secure
link only.
To set a
cookie you can write the code, as shown below:
<HTML>
<BODY>
<SCRIPT
LANGUAGE="JavaScript">
cookie_name
= "dataCookie";
var
ValueEntered;
function
setCookie() {
//Check
if cookie is already set
if(document.cookie
!= document.cookie)
{index
= document.cookie.indexOf(cookie_name);}
else
{ index
= -1;}
//
if the cookie value is not equal to the data entered by the user
if
(index == -1)
{
ValueEntered=document.myform.text1.value;
document.cookie=cookie_name+"="+ValueEntered+";
expires=Saturday, 04-Dec-2010 05:00:00
GMT";
}
alert("cookie
is set with value: "+ValueEntered);
}
</SCRIPT>
<FORM
NAME="myform">
Enter
the value for cookie: <INPUT TYPE="text" NAME="text1" size="20">
<INPUT
TYPE="button" Value="Set to Cookie"
onClick="setCookie()">
</FORM>
<?BODY
</HTML> |
The
result of the code
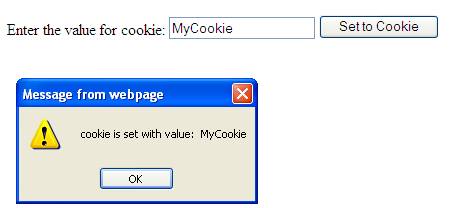
To get the
value of cookie, you need to write the function as:
function
ReadCookie(cookieName)
{
var
myCookie=""+document.cookie;
var
ind= myCookie.indexOf(cookieName);
if
(ind==-1 || cookieName=="") return "";
var
ind1= myCookie.indexOf(';',ind);
if
(ind1==-1) ind1=theCookie.length;
return
unescape(myCookie.substring(ind+cookieName.length+1,ind1));
}
|
To delete
the cookie, you need to write the function as:
function
deletecookie ( cookiename )
{
var cookiedate = new Date ( ); // current date & time
cookiedate.setTime ( cookiedate.getTime() - 1 );
document.cookie = cookiename += "=; expires=" + cookiedate.toGMTString();
} |
|